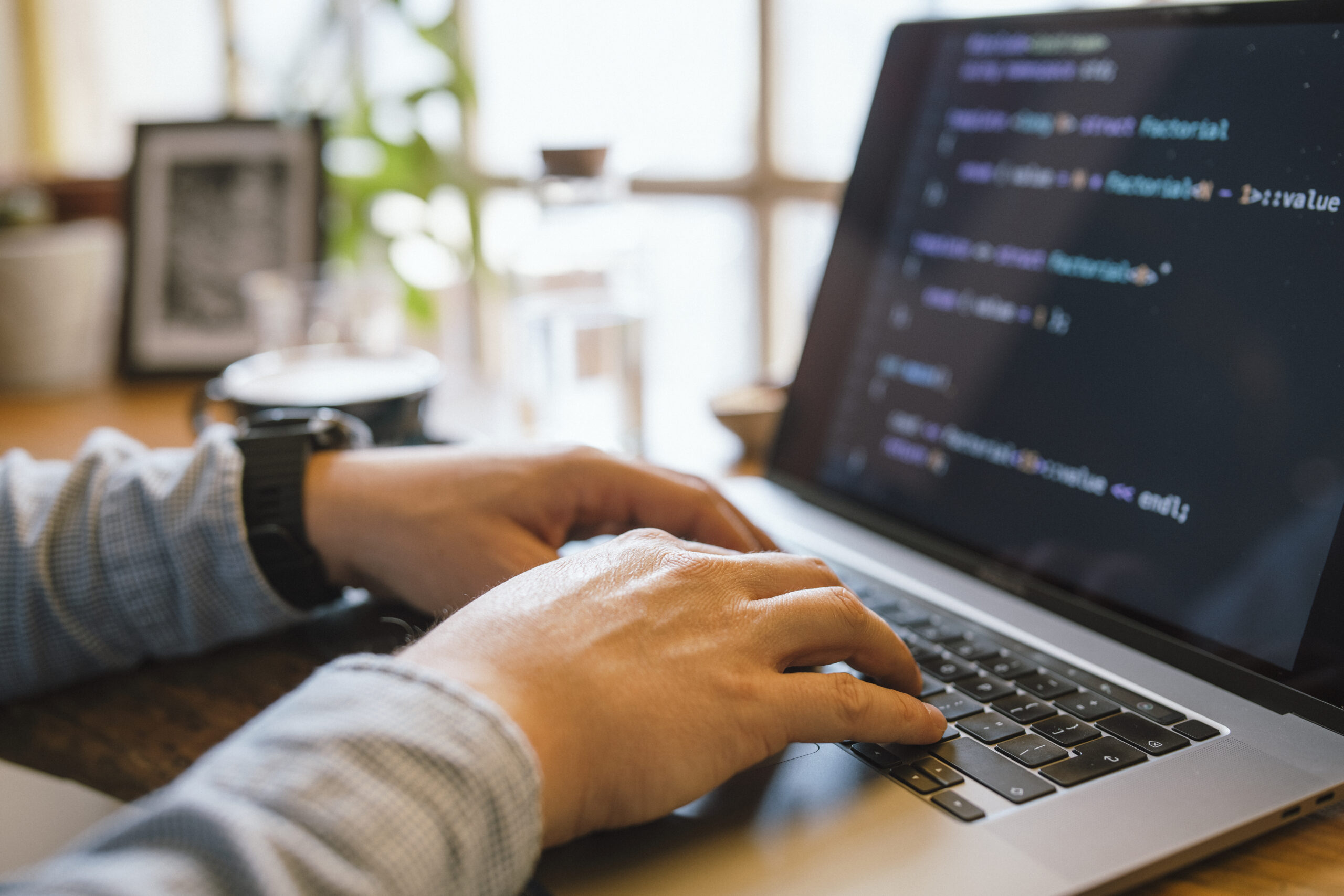
Debugging is The most essential — nevertheless generally missed — abilities inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productivity. Here are quite a few procedures to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging competencies is by mastering the instruments they use every single day. Whilst writing code is a person A part of improvement, realizing how you can interact with it effectively during execution is Similarly critical. Modern day advancement environments come equipped with highly effective debugging abilities — but many builders only scratch the area of what these instruments can perform.
Choose, one example is, an Built-in Advancement Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications let you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and also modify code on the fly. When utilised properly, they Permit you to observe particularly how your code behaves in the course of execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude developers. They let you inspect the DOM, observe network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip annoying UI issues into workable responsibilities.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage in excess of functioning processes and memory management. Mastering these tools could have a steeper Mastering curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfortable with Edition Handle devices like Git to understand code background, locate the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going over and above default configurations and shortcuts — it’s about producing an personal expertise in your development surroundings in order that when troubles occur, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you can spend resolving the particular trouble rather then fumbling through the procedure.
Reproduce the condition
One of the more significant — and infrequently forgotten — techniques in powerful debugging is reproducing the challenge. Just before jumping into the code or creating guesses, builders have to have to make a steady surroundings or scenario where the bug reliably seems. With no reproducibility, repairing a bug turns into a recreation of likelihood, frequently bringing about wasted time and fragile code adjustments.
The first step in reproducing a dilemma is collecting as much context as is possible. Request concerns like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it becomes to isolate the precise situations less than which the bug happens.
Once you’ve gathered enough data, attempt to recreate the situation in your local natural environment. This could signify inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting cases or state transitions included. These tests not merely assistance expose the trouble and also prevent regressions Later on.
From time to time, the issue could be natural environment-specific — it might come about only on sure operating methods, browsers, or beneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a move — it’s a state of mind. It needs persistence, observation, plus a methodical solution. But once you can regularly recreate the bug, you are presently halfway to fixing it. Using a reproducible circumstance, You should utilize your debugging applications extra correctly, test potential fixes safely, and communicate much more clearly with your team or end users. It turns an abstract complaint into a concrete challenge — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Completely wrong. Rather then looking at them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They frequently show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the concept very carefully and in complete. Many builders, especially when less than time strain, glance at the 1st line and quickly begin earning assumptions. But deeper in the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line number? What module or purpose triggered it? These inquiries can guide your investigation and level you towards the responsible code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and Studying to acknowledge these can greatly quicken your debugging course of action.
Some errors are vague or generic, and in All those cases, it’s critical to look at the context in which the error transpired. Check out similar log entries, input values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger challenges and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging technique starts with knowing what to log and at what amount. Popular logging concentrations involve DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic facts through progress, Data for common occasions (like successful get started-ups), Alert for prospective problems that don’t crack the appliance, ERROR for precise complications, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant information. An excessive amount of logging can obscure important messages and decelerate your procedure. Target crucial events, point out adjustments, enter/output values, and significant choice details with your code.
Format your log messages Plainly and regularly. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are achieved, and what branches of logic are executed—all with no halting This system. They’re Specifically important in manufacturing environments where by stepping by means of code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with Gustavo Woltmann AI monitoring dashboards.
Finally, wise logging is about harmony and clarity. Which has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to solution the process like a detective solving a mystery. This attitude will help stop working advanced issues into workable parts and adhere to clues logically to uncover the basis result in.
Start off by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, accumulate just as much suitable facts as you may devoid of leaping to conclusions. Use logs, take a look at scenarios, and consumer experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, sort hypotheses. Check with on your own: What may be triggering this conduct? Have any adjustments not too long ago been produced towards the codebase? Has this issue happened in advance of beneath equivalent conditions? The objective is to slender down opportunities and discover prospective culprits.
Then, test your theories systematically. Try to recreate the problem in a managed surroundings. If you suspect a selected operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, check with your code queries and let the final results lead you nearer to the reality.
Spend shut focus to small facts. Bugs usually hide while in the least predicted places—similar to a missing semicolon, an off-by-one particular error, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely understanding it. Short term fixes may cover the actual difficulty, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and assist Some others understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering hidden difficulties in complex methods.
Publish Assessments
Crafting tests is one of the most effective strategies to transform your debugging skills and General advancement effectiveness. Assessments not simply enable capture bugs early but will also serve as a safety net that gives you self-confidence when producing adjustments to the codebase. A properly-examined software is simpler to debug as it lets you pinpoint particularly wherever and when a dilemma takes place.
Get started with device assessments, which target specific features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a examination fails, you right away know in which to appear, considerably reducing some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear immediately after Formerly becoming fixed.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These assist ensure that various parts of your software perform together efficiently. They’re specifically useful for catching bugs that manifest in intricate methods with various elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect effectively, you would like to grasp its inputs, expected outputs, and edge situations. This volume of comprehension naturally qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to center on fixing the bug and observe your take a look at pass when the issue is settled. This solution ensures that the identical bug doesn’t return Sooner or later.
In short, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you catch much more bugs, more rapidly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—looking at your display for hrs, striving Option after Answer. But Just about the most underrated debugging equipment is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The problem from the new viewpoint.
When you are also close to the code for also extended, cognitive fatigue sets in. You may begin overlooking apparent errors or misreading code that you simply wrote just hours before. With this condition, your brain turns into much less effective at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique activity for 10–quarter-hour can refresh your concentration. Quite a few developers report discovering the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help protect against burnout, Specially in the course of longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away means that you can return with renewed Strength along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to move around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it surely really brings about quicker and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart method. It presents your brain Area to breathe, increases your standpoint, and aids you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It truly is a possibility to mature as being a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing important in the event you take some time to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught previously with better practices like unit tests, code reviews, or logging? The answers often expose blind places in the workflow or being familiar with and assist you Establish much better coding patterns transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. With time, you’ll start to see styles—recurring challenges or prevalent problems—which you can proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. In any case, some of the ideal builders will not be those who compose fantastic code, but people who consistently find out from their faults.
In the end, Just about every bug you repair adds a completely new layer in your talent established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging techniques takes time, follow, and endurance — but the payoff is big. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.